Discussion 2 - The Development Environment
2.1. Overview
In order for you to develop solutions to the problems that you're trying to solve, you need to have a development environment.
Here you'll get your first look at the development environment we'll be using in here, and use it to write your first code.
2.2. What is programming?
In order to function, computer hardware must be given a set of instructions— a program—to run.
Program
A program is a sequence of instructions that a computer carries out to achieve a given task.
The power of computers is that these instructions, all of them very simple, can be executed at ridiculously high speeds.
The focus of this course is to look at writing your own programs. We'll write our very first program in here in just a few minutes.
2.3. The Terminal
The terminal is a program, a type of command-line interface that we can use to operate the computer.
The terminal is not the way that most people use a computer, but it's one of the way that programmers and developers use a computer.
Terminals are what hackers use. :)
Learn about the terminal and our server here.
2.4. The Text Editor
A text editor is used to work with plain text. No fancy fonts, or underlines, or italics, or even font sizes. Just plain text. It's what many people use on the computer when they don't want to be distracted by the features of a word processor.
In our development environment, we'll be using a text editor.
You can learn more about text editors here.
2.5. The Integrated Development Environment (IDE)
Programming can be a complex process. You may be writing code in one window, testing the code in a second window, reading your email from your boss in a third window, looking up a reference in a fourth window, running a debugger in a fifth window, and... you get the idea.
It can be helpful to use a development environment where many of these windows are integrated into one piece of software.
Int this course we'll be using a very simple browser-based, integrated development environment called repl.it
(pronounced reh-plit).
2.6. The repl.it
development environment for HTML/CSS/JavaScript
The development environment for writing HTML/CSS/JavaScript (which is what we'll be doing!) has a number of different panes in the window, and they can be moved and resized as you work on your project.
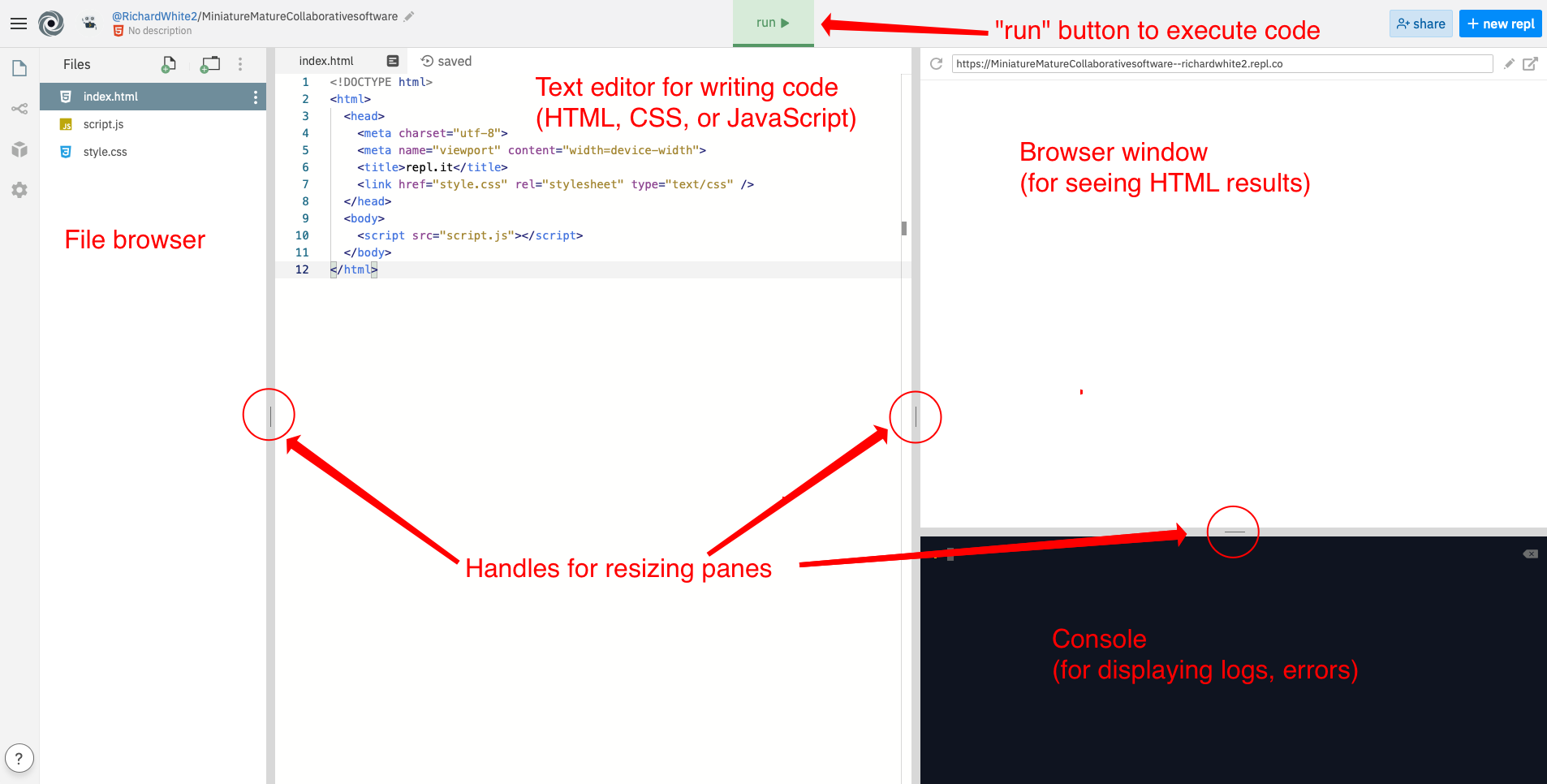
2.7. HTML, CSS, and JavaScript
This class is going to be focused on programming for the web. Let's take a few minutes to get oriented.
There are three areas of interest for us:
- HTML
- CSS
- JavaScript
2.7.1. HyperText Markup Language (HTML)
"HTML" is a type of markup that is used for presenting content. It is primarily concerned with text, which is often organized in to structures like paragraphs, headers, and lists.
We're going to become very familiar with HTML soon! For now, you can see what HTML looks like in the box there to the right. That code is the code that is used to create section 2.7 above.
2.7.2. Cascading Style Sheets (CSS)
"CSS" is a system for applying styles to the text in an HTML document. Style include things like making the text bold-faced, or in italics, or giving it a color, or putting a different line-spacing in the document. We'll be learning about CSS right after we learn about HTML.
2.7.3. JavaScript
In addition to simply displaying and styling text on a webpage, we sometimes need to be able to interact with the computer: we need to get input from the user, or calculate things, or move images around on a page, or interact with a database. For these kinds of dynamic interactions with the computer, we need a programming language.
There are lots of computer languages that you can use to give instructions to the computer, and they all have their own advantages and disadvantages. For this class, we're going to be learning a language called JavaScript. One of the reasons that JavaScript is so popular is that it is very easy to use on the World Wide Web, using a computer browser.
2.7.4. Hello World
in JavaScript
Let's write your first program in JavaScript!
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width"> <meta name="author" content="Richard White"/> <title>repl.it</title> <link href="style.css" rel="stylesheet" type="text/css" /> </head> <body> <script> // Here is the code! console.log("Hello, world!"); </script> </body> </html>
In this HTML document, everything between the <script>
tags is understood by the browser to be JavaScript code, and executed by the computer as instructions.
Personally, I like seeing output in the console, but most of the time when we're writing code, we won't want to put information there. We want to display it in the browser window.
Let's change our program so that it displays information in the browser window instead.
console.log("Hello, world!");
document.write("Hello, world!");
2.7.5. Hello World Personal
in JavaScript
An important concept in using a computer is the idea of memory, and that a computer can keep track of information over time in its memory.
Values and Variables
A value in JavaScript is often a number (like 7
or 3.14
) or a string (a string of characters, indicated by enclosing the value either in single quotes or double quotes).
A variable is a reference that can be set to indicate a given value.
Example:
This JavaScript instruction
let name = "Richard";
assigns the value Richard
to the variable name
.
Example:
This JavaScript instruction
let age = 62;
assigns the value 62
to the variable age
.
Being able to work with values and variables is central to using computers.
Trick question
A programmer put this line of code into her program:
let age = "17";
Did the programmer store a number or a string in the variable?
The value "17"
, because it has quotes around it, is a string.
A better way to write this statement would be:
let age = 17;
Another trick question
A programmer put this line of code into her program
let name = "Alice";
... but when she tried to print out a Hello message with this instruction
document.write("Hello, name!");
... it didn't work the way she thought it would.
What got printed out instead of "Hello, Alice!" ?
Because the letters in name are inside the quotes, the program printed out:
Hello, name!
The programmer probably wanted to actually print out Hello to Alice. See the next section on "concatenation" to see how to do that.
2.7.6. String concatenation
If you want to print out a series of different strings, you can link them all together in a process called concatenation. You indicate concatenation with the +
operator.
So, what gets printed if we use this JavaScript instruction?
document.write("Hello" + "name" + "!");
What gets printed if we use this JavaScript instruction?
document.write("Hello" + name + "!");
And finally, how do we get a comma and a space in there so that it reads the way it should?
2.7.7. Getting input from the user
It's nice being able to use a value stored in the variable name
, but what we really want is for the user to be able to enter any name they want, and to get a "Hello" message to that name.
Here's how you have JavaScript ask the user to enter a value:
let name = prompt("Enter your name, please!");
Once the user has entered a response, the variable name
can be used to refer to that value, just as before.