Functions and Parameters
8.0. Overview
In this session we'll be taking our first steps toward writing larger, more complex programs. We'll look at how writing procedural functions can help us better organize our code and make it less redundant, and how to write functions that uses parameters and return values to communicate with the programs that call them.
8.1. Functions
Functions
A function is simply a small program inside a larger one, whose purpose is to:
- organize your program into logical blocks of code
- reduce duplicated code (shortening and simplifying your program)
- make your program easier to maintain (by keeping your code better organized)
Generally speaking, you should include functions in your program if:
- You find that you're repeating code with minor variations
- Your program takes up more than "one screen" of space
One important strategy for reducing the amount of code you have to write is to organize code into functions.
Defining a Function
We can define functions with blocks of code that will execute when we "call" the function. As an example, here's a short (and not very useful) function to say "Hello" to somebody:
function sayHello() { document.write("Hello!"); }
These lines just define the function, but the code doesn't actually execute in our program until we call it:
sayHello()
At this point, the "Hello!" message is displayed.
8.1.1. Functions with no parameters
Here's a more realistic situation.
I'm welcoming guests to my party and I find myself saying the same thing over and over again as each guest shows up at the door. I've written a program to print what I'm saying:
let guest = "Itzy"; document.write("Welcome, " + guest + "<br />" ); document.write("Food is over there," + "<br />" ); document.write("drinks are over here," + "<br />" ); document.write("I hope you have a great time!" + "<br />" ); guest = "Gevorg"; document.write("Welcome, " + guest + "<br />" ); document.write("Food is over there," + "<br />" ); document.write("drinks are over here," + "<br />" ); document.write("I hope you have a great time!" + "<br />" ); guest = "Zahra"; document.write("Welcome, " + guest + "<br />" ); document.write("Food is over there," + "<br />" ); document.write("drinks are over here," + "<br />" ); document.write("I hope you have a great time!" + "<br />" );
This program works just great, but you can see that I've had to repeat my little speech quite a few times. We can make the program a little more efficient by writing a function to print out the parts that are repeated.
function welcome() { document.write("Food is over there," + "<br />" ); document.write("drinks are over here," + "<br />" ); document.write("I hope you have a great time!" + "<br />" ); } let guest = "Itzy"; document.write("Welcome, " + guest + "<br />" ); welcome(); guest = "Gevorg"; document.write("Welcome, " + guest + "<br />" ); welcome(); guest = "Zahra"; document.write("Welcome, " + guest + "<br />" ); welcome();
This is quite a bit more efficient than what I did before. Each time I "call" the welcome()
function in the main, the instructions in that welcome()
function are performed.
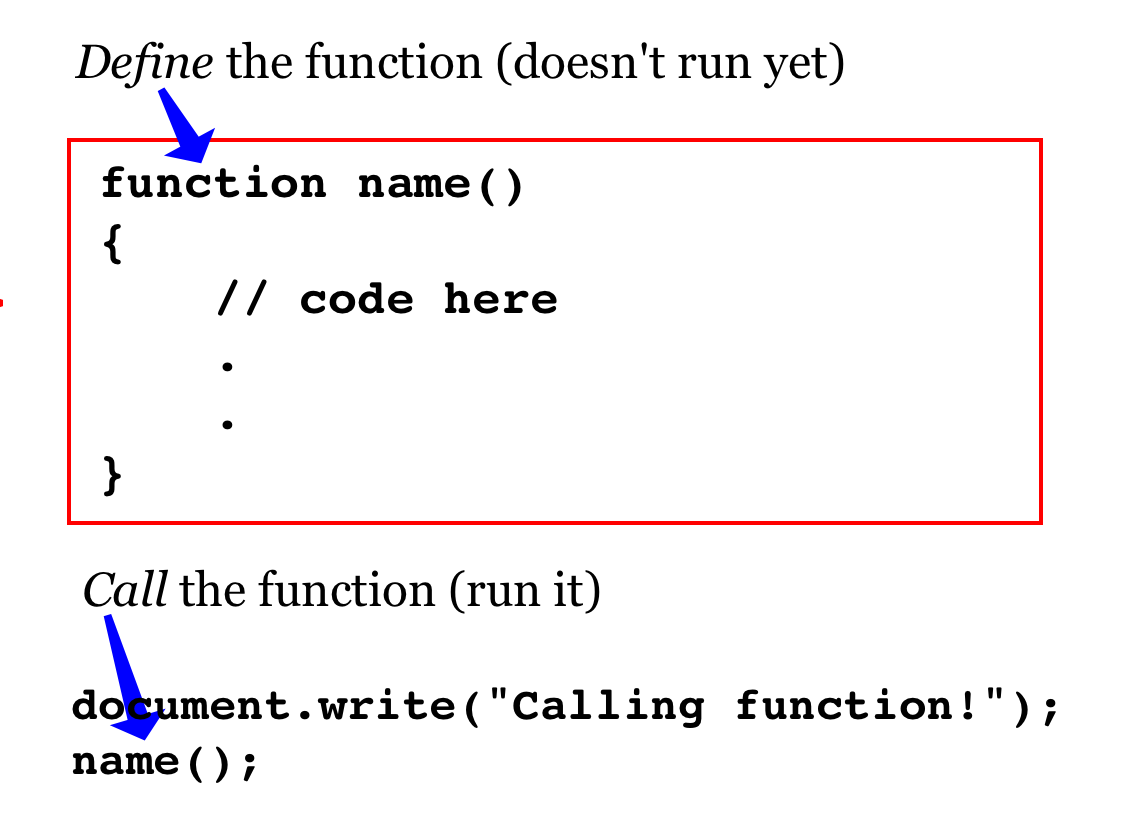
This is a good start!
To make things even a little more organized, I'm going to write another function called greetGuests
that is, in turn, going to call the welcome()
function for every guest.
This program produces exactly the same output, and includes a new function:
function welcome() { document.write("Food is over there," + "<br />" ); document.write("drinks are over here," + "<br />" ); document.write("I hope you have a great time!" + "<br />" ); } function greetGuests() { let guest = "Itzy"; document.write("Welcome, " + guest + "<br />" ); welcome(); guest = "Gevorg"; document.write("Welcome, " + guest + "<br />" ); welcome(); guest = "Zahra"; document.write("Welcome, " + guest + "<br />" ); welcome(); } // Don't forget to call the function! greetGuests()
While this might seem a little more complicated than what we had before—I have a program that calls a greetGuests()
function that in turn calls a welcome()
function—organizing code like this is very common.
8.1.2. Functions with parameters
We can make our function even more efficient by moving that print("Welcome, " + name)
instruction to somewhere inside the function. To do this, we'll just set the guest
value outside the function, and print the name out inside the function, like this. (This actually doesn't work, but it's easy to fix.)
function welcome() { document.write("Welcome, " + guest + "<br />" ); document.write("Food is over there," + "<br />" ); document.write("drinks are over here," + "<br />" ); document.write("I hope you have a great time!" + "<br />" ); } function greetGuests() { let guest = "Itzy"; welcome(); guest = "Gevorg"; welcome(); guest = "Zahra"; welcome(); } // Don't forget to call the function! greetGuests()
The issue here is that although the greetGuests()
function knows who the guest is, the welcome()
function doesn't. We need to send that bit of information in via a parameter.
The working version of the program looks like this:
function welcome(guest) { document.write("Welcome, " + guest + "<br />" ); document.write("Food is over there," + "<br />" ); document.write("drinks are over here," + "<br />" ); document.write("I hope you have a great time!" + "<br />" ); } function greetGuests() { let guest = "Itzy"; welcome(guest); guest = "Gevorg"; welcome(guest); guest = "Zahra"; welcome(guest); } // Don't forget to call the function! greetGuests()
This works just as it should!
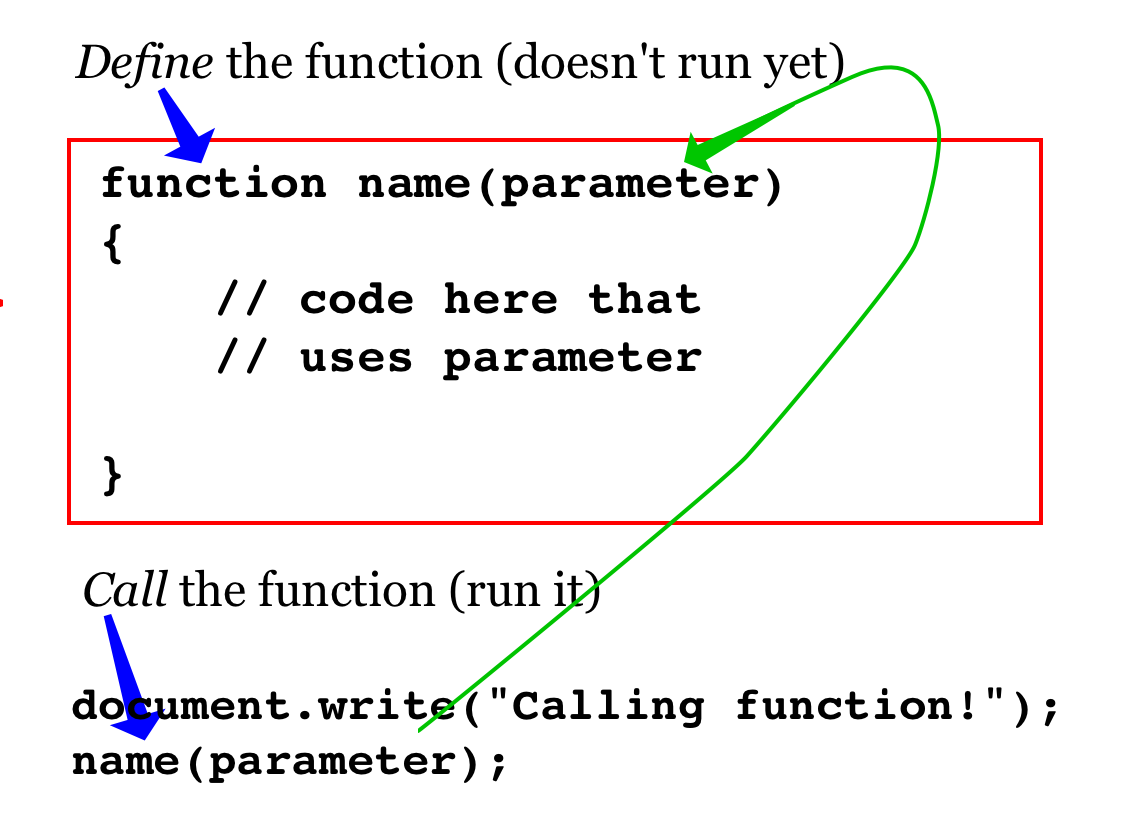
Being able to send information into a function like this is a powerful tool. We'll continue to use it throughout our study of computer science.