Unit 12: Course Review
12.0. Overview
As one comes to the end of a course, there are lots of reasons to revisit the material that has been covered. Often in the midst of a busy school year, there is a natural tendency to focus on the trees rather than the forest. As the end of the course approaches, however, one is in a better position to appreciate the larger context. Some material from the beginning of the year may even be understood better or in a different way, now that one knows a bit more about the subject.
12.1. Important Topics We've Covered
The topics covered in this class can be grouped into categories.
12.1.1. General Computer Science ideas, and Java Syntax for implementing these concepts
Most of the items in this list are also part of the AP Computer Science A curriculum.
- Binary numbers, Hexadecimal numbers
- Encapsulation
- Computational Thinking
- Output:
System.out.println()
- Input:
in.next(), in.nextInt(), in.nextDouble()
- Data Types: primitives such as
int, double, boolean
, and objects such asString
,Rectangle
- Numeric Operations, and casting to convert values
- Boolean expressions, Conditional statements:
if-else
- Writing static methods (functions) to organize code
- Iteration:
while
-loops,for
-loops - Implementing classes that represent discrete types of data
- Strings and ways of manipulating strings
- The
Array
andArrayList
for collecting lists of information - Object-oriented design principles (cohesive classes, minimizing coupling)
- Recursive algorithms
- Algorithms for sorting, searching
12.1.1. Computer and Technology Tools and Workflows
These items have to do with other aspects of "getting work done on a computer."
- Organization of files and directories in a computer file system
- Use of a Terminal/shell to interact with a file system locally
- Use of a Terminal to interact with a remote server
- Use of a text editor such as VS Code to write text-based files
- Use of an Integrated Development Environment (IDE) such as BlueJ to develop software
- Debugging strategies (print statements, IDE tester/debugger) to diagnose and fix code
- Using
git
on your local machine and GitHub to manage version control and multiple contributors.
12.1.2. Things we Haven't Covered
There are dozens of fascinating aspects of Computer Science and technology that we haven't covered in here, of course. These are all worthy of investigating, learning, understanding, mastering...
- (I got overwhelmed thinking about this list.)
12.2. Preparing for the AP Exam
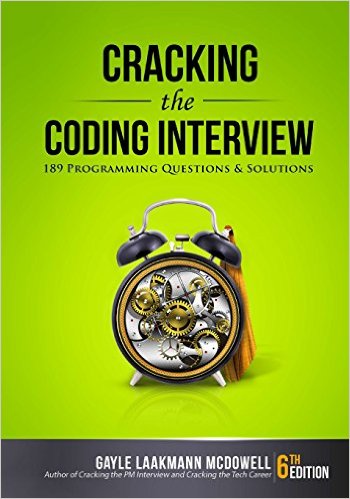
The AP Computer Science A exam will be offered Wednesday, May 7, 2025, at 12:00PM.
It's easy to adopt a cynical view towards prepping for a specific exam. An instructor risks being accused of "teaching to the test," as if that were an inappropriate strategy for reviewing material.
If it's a good test, however—and the AP Computer Science A is, in my opinion—there's nothing wrong with using it as an opportunity for a review of relevant material.
You should also be aware that the field of software development is one that very commonly tests its professionals, and preparing for those tests is a pre-interview ritual. The process of providing a demonstration of your skills and knowledge is not just an academic exercise. This is how you get a job.
With those points in mind, we're going to make sure we've got a good grasp on this material as the course comes to a close.
12.2.1. Description of the Exam
From the AP Computer Science A Course Description:
The AP Computer Science A Exam assesses student understanding of the computational thinking practices and learning objectives outlined in the course framework. The exam is 3 hours long and includes 40 multiple-choice questions and 4 free-response questions. As part of the exam, students will be given the Java Quick Reference (see Appendix), which lists accessible methods from the Java library that may be included in the exam. The details of the exam, including exam weighting and timing, can be found below:
Section Question Type Number of Questions Exam Weighting Timing I Multiple-choice questions 40 50% 90 minutes II Free-response questions 4 90 minutes Question 1: Methods and Control Structures (9 points)
- write program code to create objects of a class and call methods
- write program code to satisfy method specifications using expressions, conditional statements, and iterative statements
12.5% Question 2: Class (9 points)
- write program code to define a new type by creating a class
- write program code to satisfy method specifications using expressions, conditional statements, and iterative statements
12.5% Question 3: Array/ArrayList (9 points)
- write program code to satisfy method specifications using expressions, conditional statements, and iterative statements
- write program code to create, traverse, and manipulate elements in 1D array or ArrayList objects
12.5% Question 4: 2D Array (9 points)
- write program code to satisfy method specifications using expressions, conditional statements, and iterative statements
- write program code to create, traverse, and manipulate elements in 2D array objects
12.5%
12.2.2. Material Covered on the Exam
12.2.2.1. Multiple-Choice Questions
Multiple-choice questions in computer science can be a bit tricky, but they're a great way to quickly demonstrate your knowledge if you follow a few simple tricks. We'll discuss those.
12.2.2.2. Free-Response Questions
Historically, a variety of different types of questions have been asked.
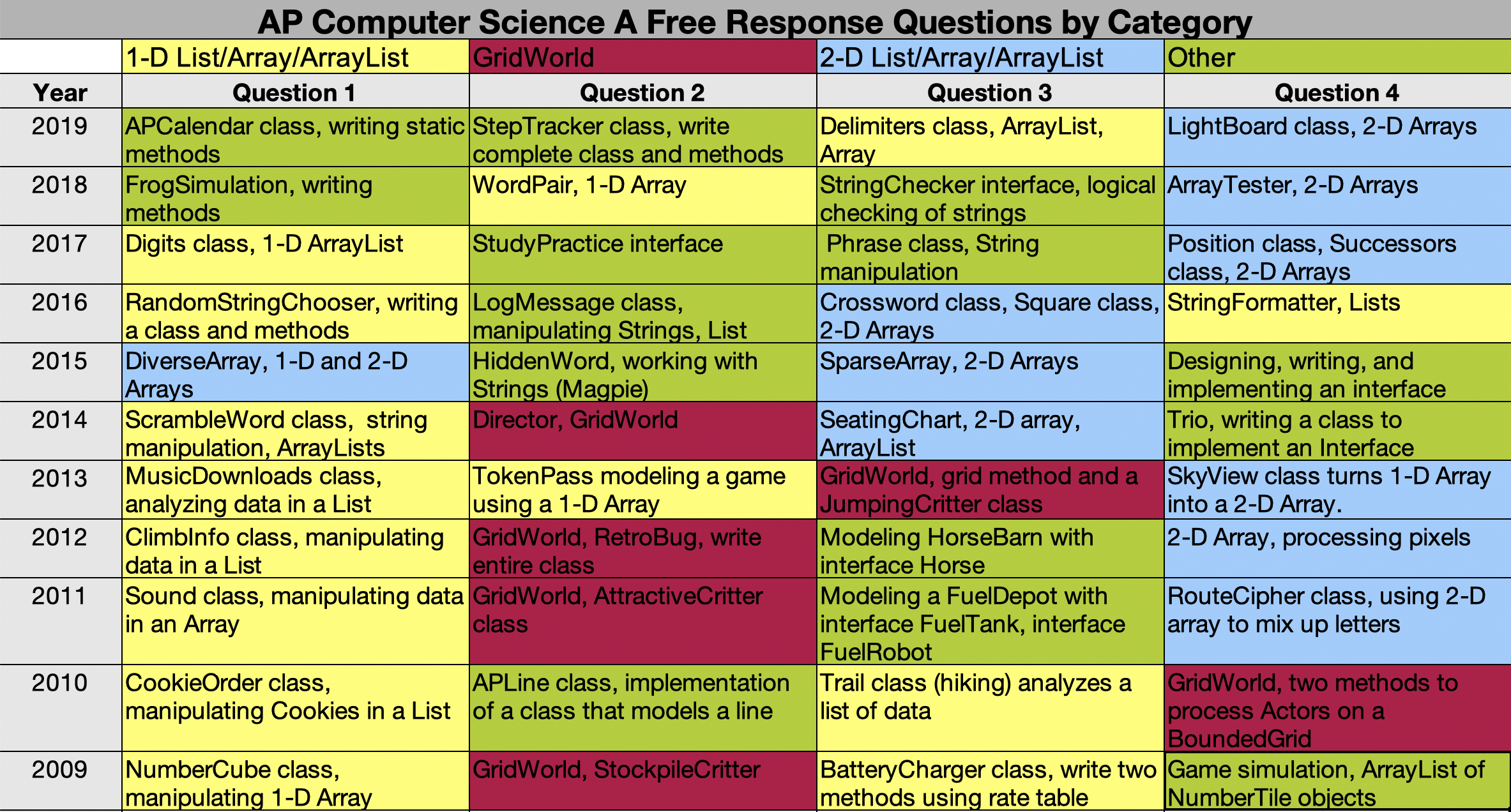
The current version of the exam includes the following question categories asked on each test.
- Question 1: Methods and Control Structures
- Question 2: Class
- Question 3: Array/ArrayList
- Question 4: 2D Array
12.2.3. Materials to use in studying for the Exam
There's no better way to learn how to solve AP problems than to... solve some actual AP problems. You've done a good job working your way through the material this year, so we just need to take time to brush up on some of the stuff that we haven't done in awhile, and remind ourselves of some of the idiosyncrasies of certain topics. And if you do find an unexpected gap in your understanding, this is the perfect chance to finally figure it out.
Free-Response Questions from Past Exams
Note that some of the questions on past exams are no longer relevant. In particular questions on GridWorld and Interfaces are no longer part of the curriculum.
- College Board: Past Exam Questions
- Skylit Solutions to Past Exams
Multiple-Choice Practice
Some people find the Multiple-Choice problems especially challenging/frustrating. For some quick practice in solving those kinds of problems, take a look at this well-organized summary of our course.
Perhaps especially useful are the practice sets of MC problems at the end of the "book".
- CSAwesome [Runestone] - Comprehensive, interactive, with practice problems.
- PracticeIt - University of Washington practice problems
- AP Computer Science A course - New Jersey Center for Teaching & Learning